Zoom
Zoom recording - starts at 15:58Links
Audio
HTML
<button type="button"
class="categoryItem"
ontouchstart="poppingClick()">
<span class="down-arrow"></span>
</button>
JavaScript
function poppingClick() {
var snd =
new Audio('sounds/tiny-button.mp3');
snd.play();
}
Video
Video courtesy of Big Buck Bunny.
With Controls
<video width="300" controls>
<source src=
"video/big_buck_bunny.mp4.crdownload"
type="video/mp4">
</video>
Autoplay
<video width="300" autoplay>
<source src=
"video/big_buck_bunny.mp4.crdownload"
type="video/mp4">
</video>
Canvas
The <canvas>
tag is a container for graphics. By default, it has no border or content. Always specify an id
, height, and width.
Example
<canvas id="myCanvas"
width="200" height="100"
style="border:1px solid #000000;">
</canvas>
Line
Strokes can be altered with ctx.strokeStyle
and ctx.lineWidth
ctx.moveTo(60,40);
ctx.lineTo(100,10);
ctx.lineTo(140,40);
ctx.lineTo(140,90);
ctx.lineTo(60,90);
ctx.lineTo(60,40);
ctx.stroke();
Circle
x | The x-coordinate of the center of the circle |
y | The y-coordinate of the center of the circle |
r | The radius of the circle |
sAngle | The starting angle, in radians (0 is at the 3 o'clock position of the arc's circle) |
eAngle | The ending angle, in radians |
counterclockwise | Optional. Specifies whether the drawing should be counterclockwise or clockwise. False is default, and indicates clockwise, while true indicates counter-clockwise |
ctx.beginPath();
ctx.arc(95, 50, 40, 0, 2 * Math.PI);
ctx.stroke();
Text
ctx.font = "28px Arial";
ctx.fillStyle = "#2a5381";
ctx.fillText("This is blue", 30, 40);
ctx.font = "55px Arial";
ctx.fillStyle = "#2a5381";
ctx.fillText("TEXT", 30, 90);
Stroke Text
ctx.font = "30px Arial";
ctx.strokeText("Hello World", 10, 30);
Linear Gradient
All grd.addColorStop
values be between 0 and 1.
x0 | The x-coordinate of the start point of the gradient |
y0 | The y-coordinate of the start point of the gradient |
x1 | The x-coordinate of the end point of the gradient |
y1 | The y-coordinate of the end point of the gradient |
var grd =
ctx.createLinearGradient(0, 50, 200, 0);
grd.addColorStop(0, "red");
grd.addColorStop(0.2, "orange");
grd.addColorStop(0.4, "yellow");
grd.addColorStop(0.6, "green");
grd.addColorStop(0.8, "blue");
grd.addColorStop(1, "purple");
// Fill with gradient
ctx.fillStyle = grd;
ctx.fillRect(10, 10, 180, 80);
Circular Gradient
x0 | The x-coordinate of the starting circle of the gradient |
y0 | The y-coordinate of the starting circle of the gradient |
r0 | The radius of the starting circle |
x1 | The x-coordinate of the ending circle of the gradient |
y1 | The y-coordinate of the ending circle of the gradient |
r1 | The radius of the ending circle |
//Create gradient
var grd =
ctx.createRadialGradient
(80, 50, 5, 90, 60, 150);
grd.addColorStop(0, "#cc0000");
grd.addColorStop(0.5, "#660000");
grd.addColorStop(1, "#654321");
// Fill with gradient
ctx.fillStyle = grd;
ctx.fillRect(10, 10, 180, 80);
Image
var img =
document.getElementById("imageForCanvas");
ctx.drawImage(img, 5, 5)
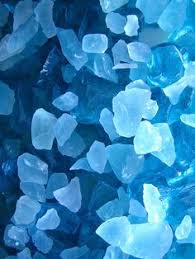