Zoom
Zoom recording - starts at 10:35Links
Single Click Order of Events
Use the following list in order of precedence for single click events. Do not do touchstart, mousedown, and/or click together. HTML5Rocks suggests using preventDefault()
inside touch
events so mouse-emulation handling doesn't occur. This does also pevent scrolling, though.
- touchstart
- touchmove
- touchend
- mouseover
- mousemove
- mousedown
- mouseup
- click
ontouchstart
This is a paragraph waiting to be touched to be changed. It can only be touched on a touch capable device like a mobile phone or tablet. Clicking it with a mouse will not accomplish anything.
function touchstart() {
var paraChange =
document.getElementById('touchstart');
paraChange.addEventListener
('touch', touchChange(event));
}
function touchChange(event) {
event.target.id = "touchStartChange";
}
touchmove
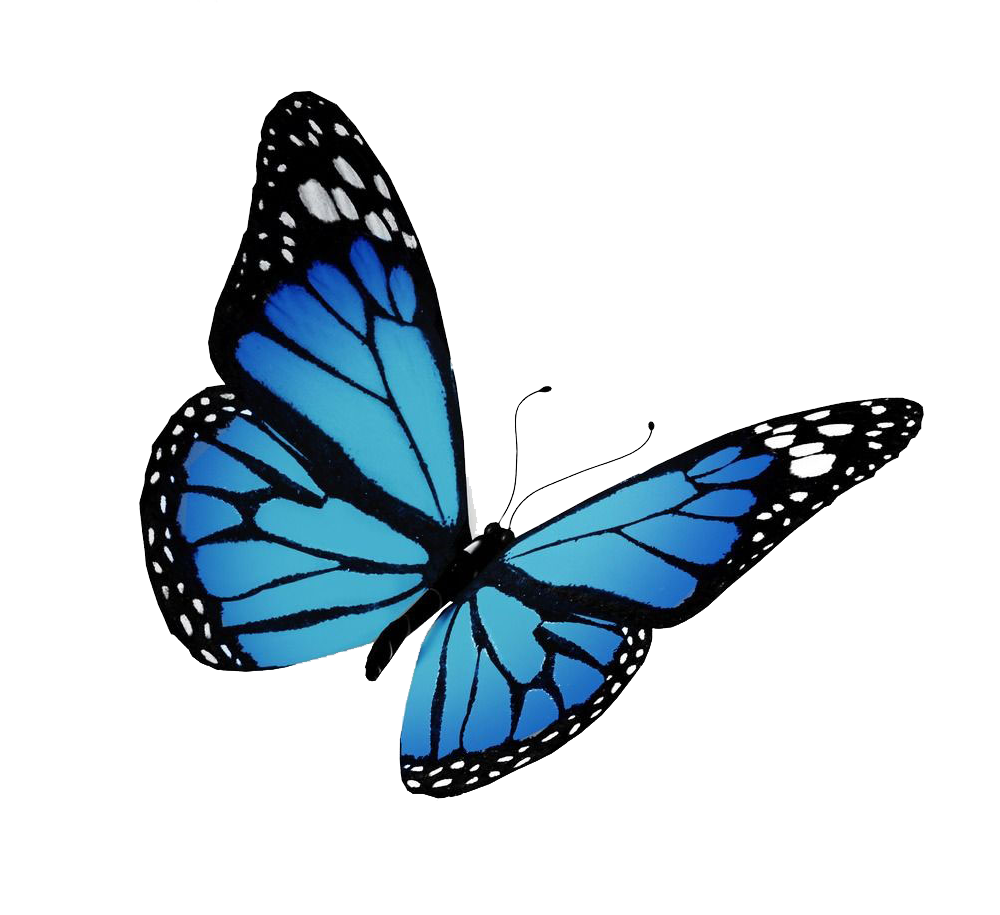
touchend
This is paragraph waiting to be changed when a touch is removed. It can only be touched on a touch capable device like a mobile phone or tablet. Clicking it with a mouse will not accomplish anything.
function touchend() {
var endChange =
document.getElementById('touchend');
endChange.addEventListener
('touchend', touchEndChange(event));
}
function touchEndChange(event) {
event.target.id = "touchEndChange";
}
click
function clickListener() {
document.getElementById('clickListener')
.addEventListener
('click', clickList(event));
console.log(event);
}
function clickList(event) {
event.target.previousSibling.textContent =
"Found it. Check the console!"
})."
}
Animation with .onload
The rolling ball animation is created using a function called with window.load
.
Rolling Ball Animation
window.onload = animateBall();
function animateBall() {
var ballContainer =
document.getElementById('ball-container');
ballContainer.addEventListener
('animationstart', start(ballContainer));
}
function start(ballContainer) {
ballContainer.classList +=
'ball-container';
var ball = document.createElement('div');
ball.classList += 'rolling-ball';
ballContainer.appendChild(ball);
}
Toggle
function toggleBox() {
var toggleBox =
document.getElementById('toggleBox');
toggleBox.classList.toggle('toggleBoxChange');
}
Transition
CSS:
#transitionBox {
width: 150px;
height: 150px;
background: maroon;
transition: width 2s;
}
#transitionBox:hover {
width: 300px;
}
#transitionEnd {
background: #3c76a6;
color: #fff;
height: 110px;
width: 300px;
padding: 20px;
}
JavaScript:
document.getElementById("transitionBox")
.addEventListener("transitionend", myFunction);
function myFunction() {
this.innerHTML = "Transitionend event occured.
The transition has completed";
this.id = 'transitionEnd';
}