Zoom
Zoom recording - starts at 21:49Loops
W3Schools states that Loops can execute a block of code a number of times. Loops are handy, if you want to run the same code over and over again, each time with a different value. This saves you from having to retype code over and over again. Both pre-test and post-test loops can be created. The pre-test has a condition at the top of a loop and the condition is evaluted before the computer executes the statements. The post-test loop evaluates the condition after the statements are run.
Five Different Kinds of Loops
Type | Purpose |
---|---|
for | loops through a block of code a number of times |
for/in | for/in - loops through the properties of an object |
for/of | for/of - loops through the values of an iterable object |
while | while - loops through a block of code while a specified condition is true |
do/while | do/while - also loops through a block of code while a specified condition is true |
For
A For loop runs through a block of code until it meets a certain condition. This is a good loop for counting.
Syntax
for (initialization; condition; update) {
statements;
}
Example
DOM Elements can be styled with a For loop. The links and headings on this page are styled with JavaScript For loops, as the example below shows.
//Change all links to a certain color
var links = document.querySelectorAll("a");
for (var i= 0; i < links.length; i++) {
links[i].style.color = "#2477CA";
}
//Change all h2 tags to a certain color
var headings = document.querySelectorAll(
"main * h2, h3, h4, h5, h6");
for (var i = 0; i < headings.length; i++) {
headings[i].style.color = "#244873";
}
For/in
Syntax
for (var in object) {
code block to be executed
}
Example
var car = {
year:"2010",
make:"Ford",
model:"Mustang"};
var text = "";
var x;
for (x in car) {
text += car[x];
}
For/of
The For/of loops lets you loop through a variety of structures including arrays, strings, maps, and nodelists. An interable is something that you can step through the properties as a collection. If you would like to display the all items from an object or array, change the = to +=.
Syntax
for (variable of iterable) {
// code block to be executed
}
Example
var cars = ['BMW', 'Volvo', 'Mini'];
var x;
for (x of cars) {
document.write(x + "
");
}
While
Syntax
while (condition) {
// code block to be executed
}
Example
var i = 1;
while (i < 3) {
window.alert(i);
i++;
}
Do/while
Do/while Loop is the only post-test loops. All others are pre-test loops. The do statements will run as long as the conditions are met for while.
Example
var i = 1;
while (i < 3) {
window.alert(i);
i++;
}
Conditional Statements
The if/else statement executes a block of code if a specified condition is true. If the condition is false, another block of code can be executed.
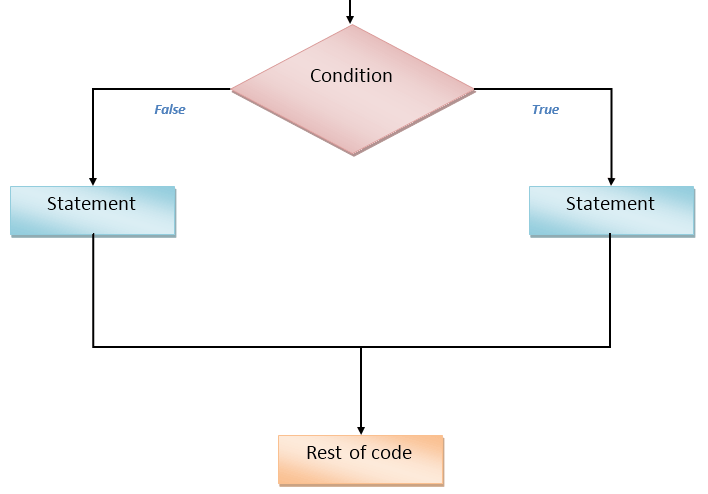
3 Different Kinds of Conditional Statements
- if statement
- if...else statement
- if...else if...else statement
Syntax
if (condition) {
// code executed if condition is true
}
else {
// code executed if condition is false
}
Example
function testNum(a) {
if (a > 0) {
return "positive";
}
else {
return "NOT positive";
}
}
console.log(testNum(-5));
// expected output: "NOT positive"
Functions
A JavaScript function is a block of code designed to perform a particular task. You can pass in arguments to a function. To call a function in a HTML page, you must include the function name plus () at the end. i.e. myFunction().
Syntax
function name(
parameter1,
parameter2,
parameter3) {
// code to be executed
}
Example
// Function called. Return value is x
var x = myFunction(4, 3);
function myFunction(a, b) {
// Function returns a times b
return a * b;
}
Variables
JavaScript variables are named containers for storing data values.
JavaScript Identifiers Naming Rules
- Names can contain letters, digits, underscores, and dollar signs.
- Names must begin with a letter, &, or _
- Names are case sensitive
- Reserved words (like JavaScript keywords) cannot be used as names
var x = 5;
var costOfMeal = $20.15
var text = document.getElementById(
'celsiusInputBox').value;
JavaScript Variable Types
Type | Purpose | Example |
---|---|---|
boolean | stores true or false | true |
number | a number with or without a decimal point | 74.3 |
string | a string of text | "San Diego" |
function | a part of a program created using the keyword function | function () {return 27;} |
object | an object such as an Array or Date | {name: "Sam", phone:"1788"} |
Example
function greetUser() {
var name = window.prompt(
"Please enter your name");
var greeting = "Hello "
+ name + ".
Welcome to my site!";
window.alert(greeting);
}
Parameters
JavaScript parameters and arguements differ in that parameters are just the name of the arguement. The arguements are the actual values. If a function is called and arguments are missing, they will be set to undefined. Always use as many parameters as you have arguements and vice versa.
function newName(
parameters 1,
parameter 2,
parameter 3) {
//statements;
}
Arrays
Declaring and Creating Arrays
Arrays are used to store multiple values together into one variable and can be strings or numbers. There are several ways to declare an array.
Declare an array:
var arrayName;
Create an array:
arrayName = new Array(size);
colors = new Array(6);
Declare and create array:
var arrayName = new Array(size);
var colors = new Array(6);
Array example:
var colors = ["Blue",
"Red",
"Green",
"Yellow"];
Accessing an Array
All values in an array are given an index number. Arrays start at 0 and count up. To access an array, you write the array name and the index number with square brackets.
Example
var colors[3];
Associative Arrays
Associative Arrays are also called a hash, map structure, or dictionaries. It is a set of key:value
pairs.
The value is stored with the key, which is used to access the value. These types of arrays look like objects when
written out. JavaScript doesn't support associative arrays and they must be referenced like an object using the name of the property instead of an index. They
return the value.
array = {key1: "value1", key2: "value2"};
var myVariable = array['key2'];